Environment Variables in Vue
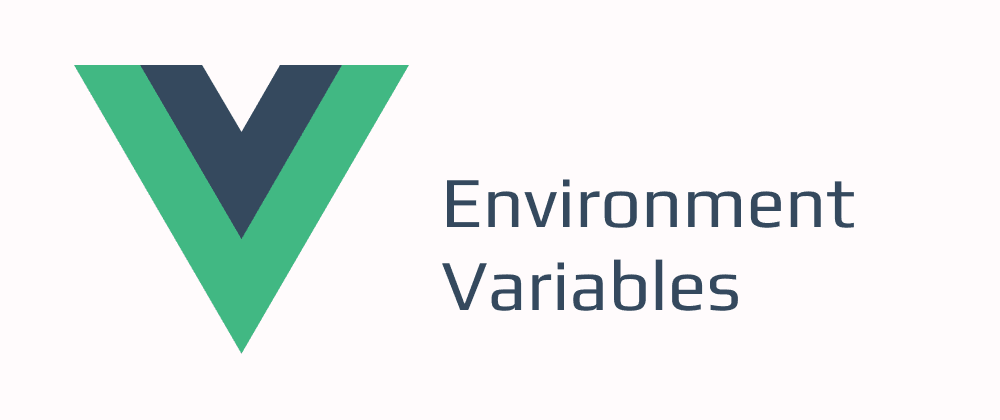
Sometimes you want your app to behave differently in local development and production. Maybe they should point to a different backend or use different API keys. Vite has very good documentation about it, Env Variables and Modes. But I will try to keep it shorter, for the use I often need.
You can create different .env
files at the root of your project.
Alternatives:
.env # loaded in all cases
.env.local # loaded in all cases, ignored by git
.env.development # vite
.env.development.local # vite, ignored by git
.env.test # vite --mode test or vite build --mode test
.env.test.local # vite --mode test or vite build --mode test, ignored by git
.env.production # vite build
.env.production.local # vite build, ignored by git
You need to make sure you have *.local
added to your .gitignore
to avoid them being checked into git, so you can keep keys you don't want to be exposed there.
The format of the environment variables is key=value
. For variables to be statically embedded into the client bundle, meaning it is available in your JavaScript code, it needs to start with VITE_
.
Examples of use cases: .env:
VITE_API_URL=https://mycoolapi.com
VITE_API_KEY=[PUBLIC_KEY]
.env.development:
VITE_API_URL=http://localhost:8080
.env.development.local:
VITE_API_KEY=[PRIVATE_KEY]
Then you can get the variables from the JavaScript code like this:
const url = import.meta.env.VITE_API_URL
That's it 😃
Remember to avoid exposing private secrets in the bundle. Even if you have it in .env.local
, it will be "somewhere" in the bundle after npm run build, though it is hard to find.